There are various ways to create squares using CSS. The easiest way is to use the width and height values. Today, we will create
squares and
rectangles using the
grid layout.
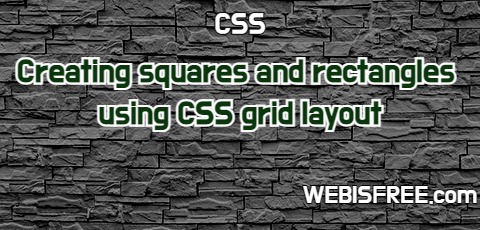
# Creating Squares Using CSS Grid-Template
To create a square or rectangle using the grid, we use the
grid-template property in CSS. This property makes it easy to create child elements as squares. It is commonly used to create multiple identical squares rather than just one.
The grid-template property is used with the following two attributes to set the width and height of child elements:
grid-template-columns
// Set the value of width
grid-template-rows
// Set the value of height
We can use values such as fr, px, and % with grid-template. Let's take a closer look at an example below.
! Example of Grid-Template Squares
Here, we will create a square with a width and height of 200px using the grid-template property.
@ Creating a Square with a Width and Height of 200pxHere is the HTML code:
<div class="grid-container">
<div class="grid-square"></div>
</div>
The following is CSS code that uses grid-template:
.grid-container {
display: grid;
grid-template-columns: 200px;
grid-template-rows: 200px;
}
.grid-square {
border: 2px solid #000;
}
When this code is executed, it appears as follows:
<div class="grid-container">
<div class="grid-square">200 x 200</div>
</div>
<style>
.grid-container {
display: grid;
grid-template-columns: 200px;
grid-template-rows: 200px;
}
.grid-square {
border: 2px solid #000;
}</style>
As you can see, we easily created a square. Now, let's create several squares of the same size:
@ Creating multiple squares of 100px by 100pxThis is a similar example but a bit different. We will create three consecutive squares that are half the size of the previous square.
This is the best way to take advantage of the grid layout's strengths.
Now, we just need to adjust the grid-template-columns and grid-template-rows values to be repeated. We can change the property values as follows:
.grid-container {
display: grid;
grid-template-columns: 100px 100px 100px;
grid-template-rows: 100px;
column-gap: 10px; // column gap of 10px
}
In the above example, we used the same 3 consecutive values for both the grid-template-columns and grid-template-rows properties. When we execute this code, it appears as follows:
<div class="grid-container-2">
<div class="grid-square">100 x 100</div>
<div class="grid-square">100 x 100</div>
<div class="grid-square">100 x 100</div>
</div>
<style>
.grid-container-2 {
display: grid;
grid-template-columns: 100px 100px 100px;
grid-template-rows: 100px;
column-gap: 10px;
}</style>
By the way, we could also use repeat() instead of repeating the values individually. In repeat(),
the 3 and 100px mean that three child elements will have the same value of 100px.
.grid-container {
display: grid;
grid-template-columns: repeat(3, 100px);
grid-template-: repeat(3, 100px);
}
As you can see, this code works the same as the previous example.
So far, we have briefly learned how to create squares and rectangles using CSS grid layout.