How to find the function name? If you always execute the same function, you can naturally know its name. However, if you have a function that receives a function as an argument and executes it, the name of the function will change. In such cases,
how can you obtain the name of the function?
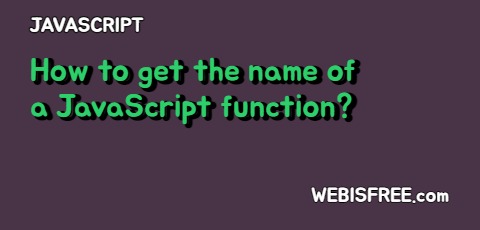
# Finding function names in JavaScript, Function name
Although it is not always necessary, there may be situations where you need to know the function name. Of course, in general, it is not common to need to know the function name. One example would be when you want to display the name of the function where an error occurred along with an error message in a specific feature.
In such a case, how can you determine the name of the function? First of all, it is possible to obtain the function name. There are three ways to do this, with a simple method based on Function.name, which was added in ES6, and two other more complex methods. Let's list the possible methods.
- Using Function.name in ES6.
- Using arguments.callee.name.
- Converting the function to a string and extracting the name using regular expressions or split().
Let's take a closer look at each method below.
! Using Function.name in ES6
Starting with ES6, you can use the name property in Function to easily obtain the function name. For example, you can write code like the following.
getFunctionName = function() {
return getFunctionName.name;
}
getFunctionName()
// Result
'getFunctionName'
As you can see, it simply returns the name of the current function.
@ Exploring other methodsNext, let's take a look at how it was done before ES6. As mentioned above, there are two more methods. However, these two methods have some constraints. Firstly, using arguments.callee.name within the function seems very simple. However, this method cannot be called in Strict mode, making it difficult to retrieve the function name in this case. For example, an error occurs in the following case.
'use strict';
getFunctionName = function() {
return arguments.callee.name;
}
The next method is to convert the function into a string and retrieve the function name contained in the string. However, this method also has constraints. In the case of functions declared as literals, the function name cannot be retrieved because they are output as function() {} without a function name.
(O) function getFunctionName() { ... }
(X) getFunctionName = function() { ... }
So far, we have briefly explored methods for retrieving function names. For this reason, it can be seen that using Function.name in ES6 is the most convenient and commonly used method.