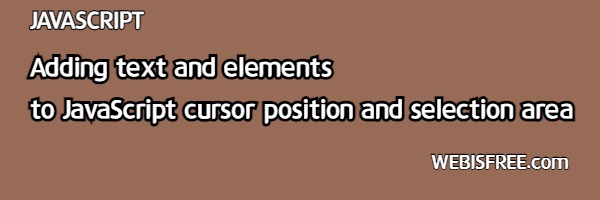
Let's learn about how to use JavaScript to add text and DOM elements at the position where the user has dragged or where the cursor is located.
# Add text or element to the position when zoomed in
Let's consider the case of creating and implementing a WYSIWYG editor from scratch. If we create a tag like "div" with an attribute of "contentEditable", how can we add elements like text or images to the cursor located inside it?
? What is the method to add to the position when it grows?...You can also find similar articles on implementing AR on Webisfree. However, today's method may be slightly different - it is to find a way to move the cursor to a different location or to a desired location even if there is no cursor.
! Understanding Example Code.
First, let's create a simple example to understand it. We added the contentEditable attribute to a div tag that allows editing to create an editable element where input is possible.
<div id="editor" contentEditable="true"></div>
(When using the contentEditable attribute, it allows for manipulation such as adding text or other contents to an element.)How do we add specific text by positioning the cursor in the corresponding area? Firstly, we use the well-known function,
getSelection().
const selection = window.getSelection();
Now the variable selection is storing the current cursor position information.
But, is it possible to change this location information arbitrarily? Of course, it is possible. The instance obtained using getSelection() has range information of the selected or cursor position within it. By changing this value to the desired value, it is possible to change it to the desired position.
range : The current node information and position index value where the cursor is located are stored.
Now, to update with a new range value, let's remove the existing range information. To do this, we will use
removeAllRanges().
selection.removeAllRanges();
The existing range value has been deleted. Next, we are trying to save new range information in the selection. To add new range information, you can use addRange(). We are trying to save the range as shown below.
const newRange = selection.getRangeAt(0); // The value of the newly saved range.
selection.addRange(newRange);
When executed as shown above, the new range information on the location is stored in the existing selection. In the example above, newRange was added, which is a variable with new location information that was previously saved using getRangeAt(0) in a different getSelection(). Although not illustrated here, it is also possible to create newRange arbitrarily, as long as there is node information to use for the location value.
@ Adding a new node to the rangeNow I want to add new text, element, or node to the changed range. As you can see below, creating a new element with
createElement() and adding it is the way to do it.
let tmpNode = document.createElement('span');
tmpNode.innerHTML = `<b>WEBISFREE</b>`;
newRange.deleteContents();
newRange.insertNode(tmpNode);
When running the above code, a new element
WEBISFREE will be added to the position of the cursor.
Observing the progress made so far may appear easy, but comprehending the complete procedure can be somewhat challenging. Essentially, the crucial process involves developing a fresh node element to include, erasing the existing range information content using the deleteContents() function, and subsequently incorporating the new node data via insertNode().
! While organizing
The most important part that needs to be re-emphasized among the above processes is as follows:
- getSelection() has range information.
- After deleting the stored range information, a new range can be added arbitrarily.
- If new elements or text are added to the range, they will be added at the desired location.You can apply this while taking note of this part. So far, we have learned the process of adding specific elements, text, etc. to the cursor position.