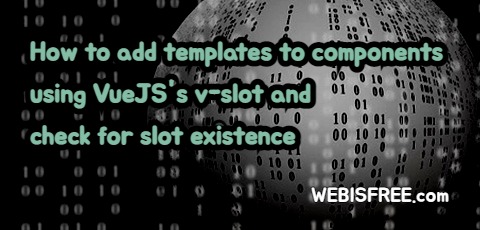
There is a way to pass data to child components in VueJS, but it is also possible to add desired templates and tags. In such cases, the commonly known method of using slot can be used. Below, we will explore how to use slot in VueJS.
# How to Pass Tags to Child Components in VueJS
According to the official website, it is possible to implement the deprecated method of using slot, but it is recommended to use v-slot. Here, we will look at how to use v-slot and how to check for the existence of slot.
@ What can be achieved with slot or v-slot?If you already know this, you can skip to the next section on using v-slot. The purpose of using slot or v-slot is a good example of when you want to add different icons to each child component. This means that it is possible to apply different icons to each one, as shown below.
<ParentComponent>
<template>
<div>
<ChildAComponent>
<i class="icon icon-arrow" />
</ChildAComponentA>
<ChildBComponent>
<i class="icon icon-close" />
</ChildBComponent>
</div>
</template>
</ParentComponent>
Here, the parent component has passed tags with different icon classes to each of the ChildA and ChildB components. The above example serves the purpose of general slot usage. In the case of
v-slot, a name can be added to the slot itself, and by using names like this, it is possible to divide and pass content in a single component like body and footer.
! How to use v-slot
Now let's take a look at how to use v-slot. When passing from a parent component to a child component, use the template tag and pass the slot name to the lower component using the v-slot attribute.
<template v-slot:(name)></template>
The name of the v-slot used here is used to distinguish where to apply the element in the child component received. You can see how it is used in the simple example below. First, when you create a v-slot with the name 'footer' in the parent element to be passed, you also need to use a template.
<div>
...
<ChildComponent>
<template v-slot:footer>
<span>webisfree.com</span>
</template>
</ChildComponent>
</div>
Now, in the ChildComponent sub-component, it will receive data through v-slot. For this, the slot name "footer" is used, corresponding to the name attribute.
<div class="footer">
...
<slot name="footer" />
</div>
Using v-slot as shown in the above example is simple. Additionally,
if you want to pass multiple v-slots to a single component, such as in addition to the footer, you can learn how to do that.
! Using multiple v-slots on a child component
When using v-slot, you can use multiple templates with different names. You can pass multiple slots (multiple slots) to child components. For example, the following is an example using body and footer.
<div>
<template v-slot:body>
<h3>Welcome to Webisfree.com</h3>
</template>
<template v-slot:footer>
<h3>Webisfree.com</h3>
</template>
</div>
We used two templates. Now, we need to divide them into body and footer and receive them in the sub-components as shown below.
<div class="content">
<div class="body">
<slot name="body" />
</div>
<div class="footer">
<slot name="footer" />
</div>
</div>
The method of use is very simple! You can now pass one or more slots.
! To check the existence of the slot:
In a sub-component, you may need to show or hide a specific tag depending on the existence of the slot. For example, if the footer class div below does not receive a slot named footer, there is no need to show it. In this case, you can apply the following method: Declare a value called isExistFooter in computed to check.
<template>
<div
v-if="isExistFooter"
class="footer"
>
<slot name="footer" />
</div>
</template>
<script>
export default {
computed: {
isExistFooter: function() {
return this.$slots.footer ? true : false;
}
},
...
}
</script>
If you look at the above example, the function isExistFooter inside computed uses this.$slots. Here, $slots allows you to access all the slots used in the component and it is also possible to access them by name as shown below.
return this.$slots.footer ? true : false;
In this way, it is possible to use this.$slots.footer to check the existence of the slot named "footer" and return a boolean value of true or false, and it is also possible to apply it to conditional statements like v-if by using it.
We have learned some ways to use v-slot in VueJS up to this point.