I recently learned about omit() in lodash. This time, let's take a look at
omitBy(), which is similar but used a little differently.
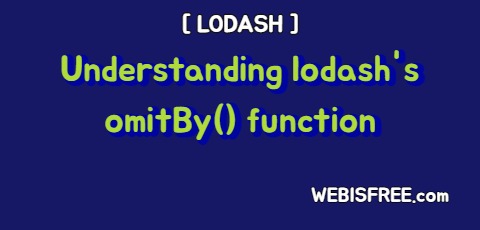
# Understanding lodash omitBy()
Like omit(), omitBy() is used to exclude properties or methods from an object. The difference is that omitBy() uses a function to specify certain conditions, rather than explicitly naming properties to exclude like in omit(). Despite this difference in usage, both methods perform the same function.
The syntax for omitBy() is simple and follows as such:
_.omitBy(targetObj, predicate)Each option is used as follows.
targetObj <Object> //
The object that is to be excluded.predicate <Function> //
Function with exclusion criteria will be included.
If the predicate function returns true in the function as a condition, the corresponding values will all be excluded.
! See an example of lodash omitBy()
We have seen what omitBy() is and briefly reviewed its syntax. Here is a simple example code using it.
@ Removing all undefined values from an objectIf you want to exclude all properties whose values are undefined, you can use omitBy() to write the code as follows.
const test = {
id: 1,
name: 'webisfree',
rank: undefined,
follow: undefined
}
_.omitBy(test, value => value === undefined)
// Result
{
id: 1,
name: 'webisfree'
}
Upon checking, it is possible to confirm that properties "rank" and "follow" which had undefined values have been removed. Note that it would be simpler to use lodash's "isUndefined" method. Now, let's try to implement the above code using _.isUndefined.
_.omitBy(test, _.isUndefined)
// Result
{
id: 1,
name: 'webisfree'
}
@ Exclude values that are not numbers."This example is for excluding the values when they are numbers, not undefined. You can write it as follows in this case."
_.omitBy(test, _.isNumber)
// Result
{
name: 'webisfree',
rank: undefined,
follow: undefined
}
From the output, we can see that the id values with numbers have been excluded.
[Note] When can we use it?omit() or omitBy() are commonly used methods. They are mainly used to remove unnecessary values and leave only the necessary values when receiving values from an API. The reason for this preprocessing process is not only because it is acceptable to not exclude, but also because the code can become more complex and unnecessary operations can be performed during the data conversion and processing process. In such cases, it can be useful to use it.
So far, we have briefly learned about the lodash method omitBy().