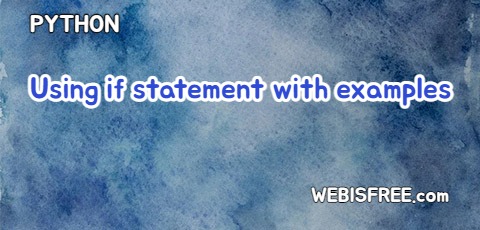
We will learn how to use if statements and examples in Python. Like other languages, Python uses a similar if syntax to branch and execute the desired syntax. Additionally, if statements are used to compare conditions as shown below.
- A == B, When the conditions A and B match or are the same
- If A != B, the two conditions do not match
Then let's take a closer look at the example below.
# Examples of using if statements in Python
If you want to check the site name as below, use it as shown below.
name = "webisfree"
if name == "webisfree":
print("True")
else:
print("False")
If you run the above example, it will print 'True' because the condition of the name variable is satisfied. If the condition is not met, it will print 'False'! Below is the case where the condition is not satisfied.
name = "webisNotfree"
if name == "webisfree":
print("True")
else:
print("False")
The "else" used above is used to enter the code to be executed when it does not meet the conditions. So let's look at ways to add other conditions.
! Adding other conditions and cases using elif.
This time, we will try to get different values depending on various situations. Please take a look at the example below.
site = 'Csite'
if site = 'Asite':
print("It is Asite")
elif site == 'Bsite'
print("It is Bsite")
elif site == 'Csite':
print("It is Csite.")
else:
print("Not found any matched site.")
This is a way to output different values based on the conditions of the Asite, Bsite, and Csite values of the site variable.
! "If statement for values containing a specific character, using the 'in' keyword."
To check if a specific character is included, use the "in" keyword as shown below. The example below checks if the value "webisfree" is included in the variable "sitename" and if it is, it executes the corresponding statement
@ test.py
sitename = 'webisfree.com'
if 'webisfree' in sitename:
print 'Okay'
# Satisfying multiple conditions using 'and' and 'or' conditions.
This time, we will learn about using the and and or conditions. There may be cases where there are multiple conditions, and in this case, it is possible to use if statements that satisfy multiple conditions using and or or. Please note that and and or should be written in lowercase letters!
! and view examples of conditions
Let's take an example of a number that is greater than 1 and less than 3.
myNum = 2
if myNum > 0 and myNum < 3:
print('True')
In the above example, we added the "and" between the two conditions to satisfy both. Now, let's look at an example using "or" for when either one satisfies.
! Learning Examples using the OR Condition
This time, it is an example of either one of the two conditions being satisfied - "or" case.
myNum = 2
if myNum > 10 and myNum < 5:
print('True')
This time, it will output True if the number is greater than 10 or less than 5. Since 2 is less than 5, it will output True in this case.