We are trying to learn about how to move elements using JavaScript.
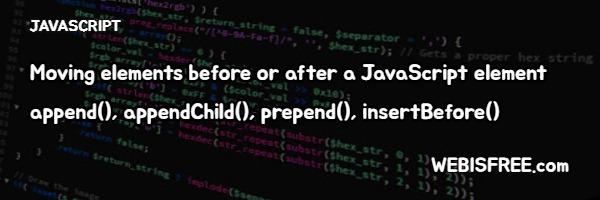
# How to Move Element Elements Using JavaScript
JavaScript has four functions to move elements as desired, as follows:
append(), appendChild()
prepend()
insertBefore()
These are functions that position the current element in front of or behind another element. Let's take a look at each of them below.
! Understanding the append() and appendChild() functions
Both functions add a specific element as a child element. The added element is positioned at the end. The two functions work similarly, but have slightly different syntax. The append() function does not work in IE 11, so
appendChild() is mostly used for compatibility. For this reason, we will discuss the appendChild() method below.
element.appendChild(Element to move)
Simple grammar rules are as shown above. Now let's create a simple example. If the following tags exist...
<body>
<div>123</div>
<p>456</p>
</body>
You can use appendChild() to add a div element inside a p element. Now let's try writing the code.
let div_ele = document.querySelector('div');
div_ele.appendChild(p_ele);
When executing the code, the div tag will move into the p tag as follows.
<body>
<p>456
<div>123</div>
</p>
</body>
We have learned about using appendChild() for moving elements up to this point.
! Understanding the JavaScript prepend() function
Following appendChild(), we will now look at the prepend() function. prepend() behaves almost identically to appendChild() in that it adds an element as a child of its parent, but the difference is that the position at which it is added is at the front, not the back.
element.prepend(...element to move)
The grammar is also almost identical. Note that prepend() does not work in IE, so please keep that in mind.
! Learning about JavaScript's insertBefore() function.
Finally, let's learn about insertBefore(). This function can also add a selected element as a child element in the same way. However, it will be positioned in front of the desired child element.
element.insertBefore(Additional elements, child elements to be moved forward)
Both of these arguments are essential elements. In addition, input is required for the child element that will move forward from here. Please note that if you add a null value, it will be added at the end.
Let's create a simple example.
<ul id="prev">
<li>1</li>
</ul>
<hr />
<ul id="next">
<li>2</li>
<li>3</li>
<li>4</li>
</ul>
<button onclick="moveToPrev()">Move</button>
If you want to add all the list elements in 'next' to 'prev', you can write the code as follows.
moveToPrev = function() {
const prev = document.querySelector('#prev');
const next = document.querySelector('#next');
prev.insertBefore(next.firstChild, prev.firstChild);
}
In other words, we have created a function that moves the first child element of 'next' to 'prev'. Check how the code works below. Note that since there is another child node in the 'li' of 'next', you need to click twice to move both.
@ Try running the code
<ul id="prev">
<li>1</li>
</ul>
<hr />
<ul id="next">
<li>2</li>
<li>3</li>
<li>4</li>
</ul>
<button onclick="moveToPrev()">Move</button>
<style>
#prev, #next { padding-left : 48px; }
#prev li, #next li { list-style: circle;}
</style>
<script>
moveToPrev = function() {
const prev = document.querySelector('#prev');
const next = document.querySelector('#next');
prev.insertBefore(next.firstChild, prev.firstChild);
}
</script>
Please make sure to check if the child elements of 'next' move correctly every time the button is pressed.
So far, we have learned several functions for moving tag elements.