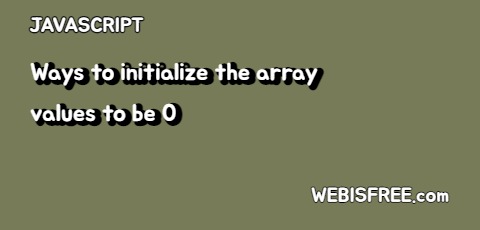
Let's find out how to initialize all array values to 0 using JavaScript.
# How to initialize all JavaScript array elements to 0
An array consists of a sequence of many values. Assuming that all values in the array are arbitrary, like the following:
let nums = [1, 3, 5, 10, 100];
How can we initialize all values in the above array to 0 now? We can use the following method.
! Use ES 6 fill()
The fill() method for arrays can easily replace or fill values with the desired value. For example, we can change all values in the array nums to 0 as shown below.
nums.fill(0);
// Outputs
[0, 0, 0, 0, 0]
The syntax of the JavaScript
fill() method is as follows.
fill(Changed value, start position, end position.)
If only the first two values are to be changed, it is entered as following.
nums.fill(0, 0, 2);
// Outputs
[0, 0, 5, 10, 100]
@ If only the array method fill() is used,
What happens if we only use fill() on an array value? In this case, all values will have the value of undefined.
nums.fill();
// Outputs
[undefined, undefined, undefined, undefined, undefined]
@ Create an array of a certain length that contains all 0 values.
The function createZeroArray() below creates an array of a certain length with values of 0.
createZeroArray = function(len) {
return new Array(len).fill(0);
}
If you want to create an array of five zeros, it would look like this.
nums = createZeroArray(5)
@ Using split() and parseFloat after converting to a string.
The following method also creates an array of 0's with the desired number.
const n = 3;
let nums = new Array(n + 1).join('0').split('').map(parseFloat)
This is a method of creating an array with the value of '0' as a string and using join(), split(), and map() simultaneously.
! Using a For loop with an array
You can also use the for loop as shown below.
let nums = new Array(5);
for (let i = 0; i < nums.length ; ++i) {
nums[i] = 0;
}
The variable 'nums' also appears initialized as an array with a value of 0.
// Outputs
[0, 0, 0, 0, 0]
We have learned how to initialize and change all values of a JavaScript array to 0 up to this point.