Previously, we learned about the JavaScript string method
startsWith(). Today, we will learn about the
endsWith() method,
which works similarly but in the opposite direction.
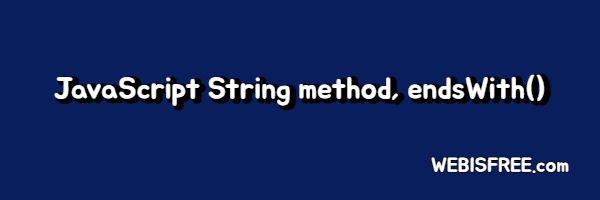
# Javascript endsWith() methods
The
endsWith() method in JavaScript is a method used on strings that
checks whether a given text matches the end of the string, starting from the end, and returns a Boolean value of true or false as a result. The basic syntax for the endsWith() method is as follows:
String.endsWith(searchText, length);//
String : Target string to check for match.
//
searchText (Required) : The string to search from the end of.
// l
ength (Option) : A value that allows setting a specific length during inspection of a target string.(Default. total length)
Sure, let's create a simple example using the endsWith() method.
! endsWith() examples
Sure, we can see how the endsWith() method behaves on each of the following strings:
const myStr = 'Hello Webisfree.com';
myStr.endsWith('Hello Webisfree.com');
myStr.endsWith('Webisfree.com');
myStr.endsWith('com');
// Results
true;
true;
true;
The results above all return true because they match from the end. Now, what if we don't distinguish between uppercase and lowercase letters? Please see below.
myStr.endsWith('webisfree.com');
myStr.endsWith('hello Webisfree.com');
// Results
false;
false;
If the capitalization is incorrect, it will return false.
[ Reference ]If you want to compare regardless of uppercase or lowercase, you can change both to either lowercase or uppercase and then compare them.
myStr.toLowerCase().endsWith('webisfree.com'.toLowerCase());
// Results
true;
@ Use endsWith() by setting length as the second argument.Next, let's discuss setting the length. What would be the result of the following?
'webisfree'.endsWith('free', 9);
'webisfree'.endsWith('free', 8);
// Results
true;
false;
The length at the end determines the length of the string to be compared. In the first example, the length of 9 is used for the entire string 'webisfree', so it checks if 'free' is at the end of the string and returns true. However, when the length is 8, it compares only 'webisfre', which doesn't match 'free'. Of course, if it were 'fre', then it would match.
'webisfree'.endsWith('fre', 8);
// Results
true;
Additionally, how is the return value determined in cases where the compared value is empty?
myStr.endsWith('');
// Result
true;
The result mentioned above is true. However, in the cases below, they will all return false.
myStr.endsWith();
myStr.endsWith(null);
myStr.endsWith(undefined);
// All Results
false;
So far, we have learned about the string method endsWith().